<div class="button"><span>hover me to change</span></div>
.button {
margin:40px auto;
width:200px;
height:60px;
padding:0 30px;
line-height: 60px;
text-align: center;
position: relative;
appearance: none;
background: #f72359;
border: none;
color: white;
font-size: 1.2em;
cursor: pointer;
outline: none;
overflow: hidden;
border-radius: 100px;
}
.button span {
position: relative;
}
.button::before {
--size: 0;
content: '';
position: absolute;
left: var(--x);
top: var(--y);
width: var(--size);
height: var(--size);
background: radial-gradient(circle closest-side, #4405f7, transparent);
transform: translate(-50%, -50%);
transition: width .2s ease, height .2s ease;
}
.button:hover::before {
--size: 400px;
}
document.querySelector('.button').addEventListener('mousemove', function (e) {
const x = e.pageX - this.offsetLeft
const y = e.pageY - this.offsetTop
this.style.setProperty('--x', `${ x }px`)
this.style.setProperty('--y', `${ y }px`)
})
# css变量
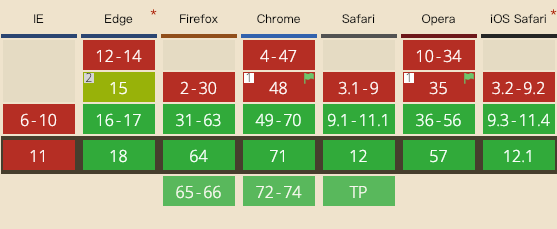
# 一般用法
我们要先用--*来定义一个变量,并设置好内容,然后再利用var取出内容,和js里var用法不同,css中的var是调取而不是定义。
div{/*放在自己的属性上,自己用*/
--size:10px;
font-size:var(--size);
}
变量可以在上层定义,下层使用。
:root{//上层定义--size
--size:10px;
}
div{//下层使用--size
font-size:var(--size);
}
# 变量不存在/变量格式错误
var的后面还可以跟一个备用属性,例如color:var(--color,blue),这时说当--color找不到的时候,用blue代替。
如果想跟两个变量怎么办?var(--color,var(--color1,blue))。注意不能写成var(--color,--color1,blue)。
如果变量存在,但是格式不对是不会用备用的。例如--color:10px,这样只会导致没有效果,但是不会用后面的备用属性。
# 与calc结合使用
:root{
--size:10;
}
div{
font-size:calc(1px * var(--size));
}
# js操作var属性
由于var属性的特殊性,因此只能用getPropertyValue和setProperty来获取/设置了。
//获取行内样式上style的var属性
element.style.getPropertyValue("--size");
//获取css中的style的var属性
getComputedStyle(element).getPropertyValue("--size");
//设置行内样式style上的var属性
element.style.setProperty("--size", 20);
← 基础知多少